Standard code style in project development is not only baneficial for others to read, but also for later code maintenance and code refactoring. So standard code style is very import for developing large projects. Next, I will briefly introduce the python code style guide EPE8, hoping to help you.
缩进
使用 4 个空格进行代码缩进,绝对禁止制表符和空格的混用1
2
3
4
5
6
7
8
9
10
11
12
13
14# Aligned with opening delimiter.
foo = long_function_name(var_one, var_two,
var_three, var_four)
# Add 4 spaces (an extra level of indentation) to distinguish arguments from the rest.
def long_function_name(
var_one, var_two, var_three,
var_four):
print(var_one)
# Hanging indents should add a level.
foo = long_function_name(
var_one, var_two,
var_three, var_four)
字符数
每行最多不要超过 79 字符1
2
3with open('/path/to/some/file/you/want/to/read') as file_1, \
open('/path/to/some/file/being/written', 'w') as file_2:
file_2.write(file_1.read())
换行
如果需要换行的话在二元运算符前面换行,如1
2
3
4
5income = (gross_wages
+ taxable_interest
+ (dividends - qualified_dividends)
- ira_deduction
- student_loan_interest)
空行
顶级函数和类定义之间使用2个空行,类内部各个方法之间使用1个空行。
为了让有关联的函数成组,可以在各函数组之间有节制地添加空格来区分各个逻辑部分。相互关联的一组单行函数之间可以省略空格
编码
程序编码使用 UTF-8
导入
导入语句通常单独成行1
2import os
import sys
不能写成1
import os, sys
但是像下面这样写是可以的1
from subprocess import Popen, PIPE
导入语句应按照以下顺序进行分组
- 标准库的导入
- 相关第三方库的导入
- 本地应用程序/库——特定库的导入
每组导入之间加一个空行,通常建议所有的导入都使用绝对路径
__all__
, __author__
, __version__
等这样的模块级“呆名“(也就是名字里有两个前缀下划线和两个后缀下划线),应该放在文档字符串的后面,以及除 from __future__
之外的import表达式前面
空格
- 紧靠小括号、中括号或大括号内部禁止使用空格
1
2Yes: spam(ham[1], {eggs: 2})
No: spam( ham[ 1 ], { eggs: 2 } ) - 紧挨着逗号、分号或冒号之前禁止使用空格
1
2Yes: if x == 4: print x, y; x, y = y, x
No: if x == 4 : print x , y ; x , y = y , x - 紧挨着函数参数列表的左括号之前禁止使用空格
1
2Yes: spam(1)
No: spam (1) - 紧挨着索引或切片操作的左括号之前禁止使用空格
1
2Yes: dct['key'] = lst[index]
No: dct ['key'] = lst [index] - 没必要为了与另一条语句对齐而在运算符两边使用多个空格
1
2
3
4
5
6
7Yes: x = 1
y = 2
long_variable = 3
No: x = 1
y = 2
long_variable = 3 - 始终在以下二元操作符两侧各放1个空格:赋值(
=
)、增量赋值(+=
,-=
)、比较(==
,<
,in
,not
,is
,is not
等)、布尔(and
,or
,not
) - 在数学运算符两侧添加空格
- 用于指定关键字参数或默认参数值时,请勿在
=
两侧使用空格1
2
3
4
5Yes: def complex(real, imag=0.0):
return magic(r=real, i=imag)
No: def complex(real, imag = 0.0):
return magic(r = real, i = imag) - 通常不鼓励在同一行放置多条语句
- 避免在行尾使用空格
注释
注释一定要和代码一致,更改代码之前先更改注释,这是一个好习惯。注释结束应该有一个
。
,且首字母大写。块注释通常适用于跟随它们的某些(或全部)代码,并缩进到与代码相同的级别。块注释的每一行开头使用一个#和一个空格(除非块注释内部缩进文本)。
块注释内部的段落通常只有一个#的空行分隔。
有节制的使用行内注释,因为这会分散注意力
命名
模块应该用简短全小写的名字,如果为了提升可读性,下划线也是可以用的。Python包名也应该使用简短全小写的名字,但不建议用下划线。
类名一般使用首字母大写的约定。
注意,对于内置的变量命名有一个单独的约定:大部分内置变量是单个单词(或者两个单词连接在一起),首字母大写的命名法只用于异常名或者内部的常量。
函数名应该小写,如果想提高可读性可以用下划线分隔。
始终要将 self 作为实例方法的的第一个参数,始终要将 cls 作为类静态方法的第一个参数。
如果函数的参数名和已有的关键词冲突,在最后加单一下划线比缩写或随意拼写更好。因此 class_ 比 clss 更好。
使用下划线分隔小写单词以提高可读性,建议使用这样的函数命名规则
常量通常定义在模块级,通过下划线分隔的全大写字母命名。例如: MAX_OVERFLOW 和 TOTAL。
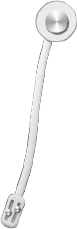
...
...
If you like this blog or find it useful for you, you are welcome to comment on it. You are also welcome to share this blog, so that more people can participate in it. If the images used in the blog infringe your copyright, please contact the author to delete them. Thank you !